On my journey to becoming a web developer in Japan, I have found two concepts difficult to understand. The first, is higher order functions. The second is recursion.
What is recursion?
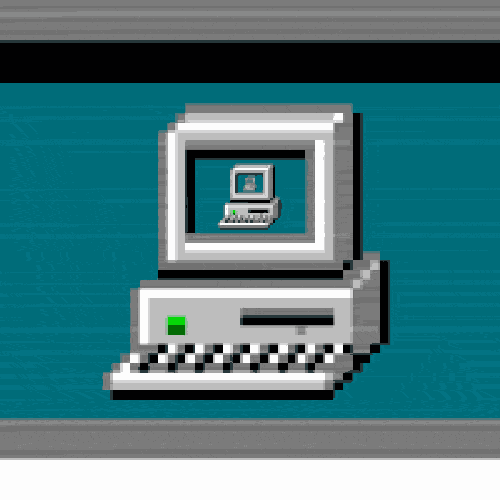
Recursion (adjective: recursive) occurs when a thing is defined in terms of itself or of its type… a function being defined is applied within its own definition. While this apparently defines an infinite number of instances (function values), it is often done in such a way that no infinite loop or infinite chain of references can occur. - Wikipedia
Now, in layman’s terms
Recursion is when the function uses itself in a loop until some test, defined within itself, is met. - Zach Stone
Let’s look at an example.
Define a function that takes an input number. The program console logs the number and every subsequent number reducing by one each time. It does this until it reaches 0. When the function reaches 0, it should console log “I’m finished.”
without recursion (iteratively)
const countDown = (num) => {
for (let x = num; x >= 0; x--) {
if (x === 0) {
console.log("I'm finished");
} else {
console.log(x);
}
}
};
countDown(20); // 20 , 19, 18, 17, 16, 15 ,14 ,13 ,12 ,11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, "I'm finished"
with recursion (recursively)
The key is thinking about the base case. That’s the test to see if we can end the loop.
The base case in this situation is
if (x === 0)
So we need to rewrite the function.
const countDown = (num) => {
if (num === 0) {
console.log("I'm finished");
} else {
console.log(num);
return countDown(num - 1);
}
};
countDown(20); // 20 , 19, 18, 17, 16, 15 ,14 ,13 ,12 ,11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, "I'm finished"
We did it!!!!
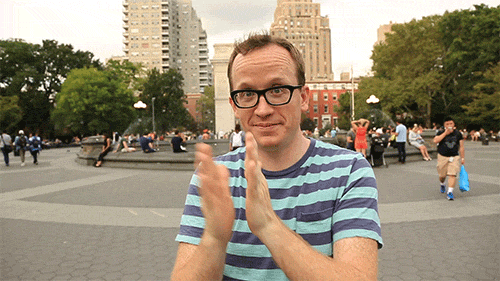
The function calls itself over and over, reducing by 1 each time. Finally, when the base case is met, it console logs “I’m finished” and ends.
Here are some resources to learn more
- Recursion in 100 Seconds <3
- A great intro to recursion by Colte Steele
- Codewars playlist of recursion problems
- freeCodeCamp article
- freeCodeCamp YouTube vid
Did you enjoy this article? Or did I get something wrong?
Feel free to contact me using my website
Have a fantastic day!